saalek110
Well-Known Member
opengl
یک برنامه در اوایل تاپیک از سایت زیر:
A simple example of using an Android Renderer to illustrate OpenGL ES boilerplate.
با کد زیر:
پست شد که بهتره در 3 فایل تقسیم بشود. دو کلاس داخل کد هست که ببرید به فایلهای جاوای جدید.
مکعب چرخان:
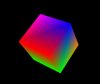
در oncreate کلاس اصلی :
همان طور که می بینید پنجره جدید تنظیم شده.
در کلاس DemoRenderer که از Renderer مشتق شده...
ابتدا یک آبجکت از کلاس مکعب ساخته شده:
و بعد یک متغیر float برای چرخش مکعب ایجاد شده.
و بعد 3 تابع داریم.
onSurfaceCreated و onSurfaceChanged و onDrawFrame
در مورد اولی:
منبع می گوید:
In onSurfaceCreated, you initialize your program and your initial configurations.
This method is called once for each Surface’s view’s cycle.
But the Surface can be destroyed and this method will be called when the next one is created.
متد اولی یعنی onSurfaceCreated طبق گفته سایت بالا فقط یک بار اجرا میشه. برخلاف سومی یعنی onDrawFrame که گفته very often اجرا میشه. یعنی خیلی زیاد.
در سومین متد یعنی onDrawFrame می بینید متد Draw مکعب فراخوانی شده.
تابع glTranslatef که قبل رسم مکعب آمده برای تغییر مکان شکل به جای مناسب است.
و glRotatef برای چرخاندن شکل است.
در خط آخر متد onDrawFrame داریم:
و در تابع چرخش داشتیم:
مقدار متغیر چرخش مدام در حال تغییر است چون متد onDrawFrame مدام اجرا می شود.
پس مکعب مدام در حال چرخش خواهد بود.
یک برنامه در اوایل تاپیک از سایت زیر:
A simple example of using an Android Renderer to illustrate OpenGL ES boilerplate.
با کد زیر:
JavaScript:
/* GraphicGlDemoActivity.java
* Author: Yong Bakos
* Since: 11/26/2012
* Thanks to:
* Cube: http://intransitione.com/blog/create-a-spinning-cube-with-opengl-es-and-android/
* OpenGL Boilerplate: http://www.jayway.com/2009/12/03/opengl-es-tutorial-for-android-part-i/
*/
package com.humanoriented.sudoku;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.nio.FloatBuffer;
import javax.microedition.khronos.egl.EGLConfig;
import javax.microedition.khronos.opengles.GL10;
import android.app.Activity;
import android.opengl.GLSurfaceView;
import android.opengl.GLSurfaceView.Renderer;
import android.opengl.GLU;
import android.os.Bundle;
import android.view.Window;
import android.view.WindowManager;
public class GraphicGlDemoActivity extends Activity {
public class DemoRenderer implements Renderer {
private Cube cube = new Cube();
private float rotation;
@Override
public void onSurfaceCreated(GL10 gl, EGLConfig config) {
gl.glClearColor(0.0f, 0.0f, 0.0f, 0.5f);
// Depth buffer setup.
gl.glClearDepthf(1.0f);
// Enables depth testing.
gl.glEnable(GL10.GL_DEPTH_TEST);
// The type of depth testing to do.
gl.glDepthFunc(GL10.GL_LEQUAL);
// Really nice perspective calculations.
gl.glHint(GL10.GL_PERSPECTIVE_CORRECTION_HINT, GL10.GL_NICEST);
}
@Override
public void onSurfaceChanged(GL10 gl, int width, int height) {
// Sets the current view port to the new size.
gl.glViewport(0, 0, width, height);
// Select the projection matrix
gl.glMatrixMode(GL10.GL_PROJECTION);
// Reset the projection matrix
gl.glLoadIdentity();
// Calculate the aspect ratio of the window
GLU.gluPerspective(gl, 45.0f, (float) width / (float) height, 0.1f, 100.0f);
// Select the modelview matrix
gl.glMatrixMode(GL10.GL_MODELVIEW);
// Reset the modelview matrix
gl.glLoadIdentity();
}
@Override
public void onDrawFrame(GL10 gl) {
gl.glClear(GL10.GL_COLOR_BUFFER_BIT | GL10.GL_DEPTH_BUFFER_BIT);
gl.glLoadIdentity();
gl.glTranslatef(0.0f, 0.0f, -10.0f);
gl.glRotatef(rotation, 1.0f, 1.0f, 1.0f);
cube.draw(gl);
gl.glLoadIdentity();
rotation -= 0.15f;
}
}
class Cube {
private FloatBuffer mVertexBuffer;
private FloatBuffer mColorBuffer;
private ByteBuffer mIndexBuffer;
private float vertices[] = {
-1.0f, -1.0f, -1.0f,
1.0f, -1.0f, -1.0f,
1.0f, 1.0f, -1.0f,
-1.0f, 1.0f, -1.0f,
-1.0f, -1.0f, 1.0f,
1.0f, -1.0f, 1.0f,
1.0f, 1.0f, 1.0f,
-1.0f, 1.0f, 1.0f
};
private float colors[] = {
0.0f, 1.0f, 0.0f, 1.0f,
0.0f, 1.0f, 0.0f, 1.0f,
1.0f, 0.5f, 0.0f, 1.0f,
1.0f, 0.5f, 0.0f, 1.0f,
1.0f, 0.0f, 0.0f, 1.0f,
1.0f, 0.0f, 0.0f, 1.0f,
0.0f, 0.0f, 1.0f, 1.0f,
1.0f, 0.0f, 1.0f, 1.0f
};
private byte indices[] = {
0, 4, 5, 0, 5, 1,
1, 5, 6, 1, 6, 2,
2, 6, 7, 2, 7, 3,
3, 7, 4, 3, 4, 0,
4, 7, 6, 4, 6, 5,
3, 0, 1, 3, 1, 2
};
public Cube() {
ByteBuffer byteBuf = ByteBuffer.allocateDirect(vertices.length * 4);
byteBuf.order(ByteOrder.nativeOrder());
mVertexBuffer = byteBuf.asFloatBuffer();
mVertexBuffer.put(vertices);
mVertexBuffer.position(0);
byteBuf = ByteBuffer.allocateDirect(colors.length * 4);
byteBuf.order(ByteOrder.nativeOrder());
mColorBuffer = byteBuf.asFloatBuffer();
mColorBuffer.put(colors);
mColorBuffer.position(0);
mIndexBuffer = ByteBuffer.allocateDirect(indices.length);
mIndexBuffer.put(indices);
mIndexBuffer.position(0);
}
public void draw(GL10 gl) {
gl.glFrontFace(GL10.GL_CW);
gl.glVertexPointer(3, GL10.GL_FLOAT, 0, mVertexBuffer);
gl.glColorPointer(4, GL10.GL_FLOAT, 0, mColorBuffer);
gl.glEnableClientState(GL10.GL_VERTEX_ARRAY);
gl.glEnableClientState(GL10.GL_COLOR_ARRAY);
gl.glDrawElements(GL10.GL_TRIANGLES, 36, GL10.GL_UNSIGNED_BYTE,
mIndexBuffer);
gl.glDisableClientState(GL10.GL_VERTEX_ARRAY);
gl.glDisableClientState(GL10.GL_COLOR_ARRAY);
}
}
@Override
public void onCreate(Bundle state) {
super.onCreate(state);
requestWindowFeature(Window.FEATURE_NO_TITLE);
getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN, WindowManager.LayoutParams.FLAG_FULLSCREEN);
GLSurfaceView view = new GLSurfaceView(this);
view.setRenderer(new DemoRenderer());
setContentView(view);
}
}
مکعب چرخان:
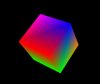
در oncreate کلاس اصلی :
JavaScript:
@Override
protected void onCreate(Bundle state) { //Bundle savedInstanceState
// super.onCreate(savedInstanceState);
// setContentView(R.layout.activity_main);
super.onCreate(state);
requestWindowFeature(Window.FEATURE_NO_TITLE);
getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN, WindowManager.LayoutParams.FLAG_FULLSCREEN);
GLSurfaceView view = new GLSurfaceView(this);
view.setRenderer(new DemoRenderer());
setContentView(view);
}
در کلاس DemoRenderer که از Renderer مشتق شده...
JavaScript:
public class DemoRenderer implements Renderer {
private Cube cube = new Cube();
private float rotation;
@Override
public void onSurfaceCreated(GL10 gl, EGLConfig config) {
gl.glClearColor(0.0f, 0.0f, 0.0f, 0.5f);
// Depth buffer setup.
gl.glClearDepthf(1.0f);
// Enables depth testing.
gl.glEnable(GL10.GL_DEPTH_TEST);
// The type of depth testing to do.
gl.glDepthFunc(GL10.GL_LEQUAL);
// Really nice perspective calculations.
gl.glHint(GL10.GL_PERSPECTIVE_CORRECTION_HINT, GL10.GL_NICEST);
}
@Override
public void onSurfaceChanged(GL10 gl, int width, int height) {
// Sets the current view port to the new size.
gl.glViewport(0, 0, width, height);
// Select the projection matrix
gl.glMatrixMode(GL10.GL_PROJECTION);
// Reset the projection matrix
gl.glLoadIdentity();
// Calculate the aspect ratio of the window
GLU.gluPerspective(gl, 45.0f, (float) width / (float) height, 0.1f, 100.0f);
// Select the modelview matrix
gl.glMatrixMode(GL10.GL_MODELVIEW);
// Reset the modelview matrix
gl.glLoadIdentity();
}
@Override
public void onDrawFrame(GL10 gl) {
gl.glClear(GL10.GL_COLOR_BUFFER_BIT | GL10.GL_DEPTH_BUFFER_BIT);
gl.glLoadIdentity();
gl.glTranslatef(0.0f, 0.0f, -10.0f);
gl.glRotatef(rotation, 1.0f, 1.0f, 1.0f);
cube.draw(gl);
gl.glLoadIdentity();
rotation -= 0.15f;
}
}//class DemoRenderer
JavaScript:
private Cube cube = new Cube();
JavaScript:
private float rotation;
onSurfaceCreated و onSurfaceChanged و onDrawFrame
در مورد اولی:
منبع می گوید:
In onSurfaceCreated, you initialize your program and your initial configurations.
This method is called once for each Surface’s view’s cycle.
But the Surface can be destroyed and this method will be called when the next one is created.
متد اولی یعنی onSurfaceCreated طبق گفته سایت بالا فقط یک بار اجرا میشه. برخلاف سومی یعنی onDrawFrame که گفته very often اجرا میشه. یعنی خیلی زیاد.
در سومین متد یعنی onDrawFrame می بینید متد Draw مکعب فراخوانی شده.
JavaScript:
@Override
public void onDrawFrame(GL10 gl) {
gl.glClear(GL10.GL_COLOR_BUFFER_BIT | GL10.GL_DEPTH_BUFFER_BIT);
gl.glLoadIdentity();
gl.glTranslatef(0.0f, 0.0f, -10.0f);
gl.glRotatef(rotation, 1.0f, 1.0f, 1.0f);
cube.draw(gl);
gl.glLoadIdentity();
rotation -= 0.15f;
}
JavaScript:
cube.draw(gl);
و glRotatef برای چرخاندن شکل است.
در خط آخر متد onDrawFrame داریم:
JavaScript:
rotation -= 0.15f;
JavaScript:
gl.glRotatef(rotation, 1.0f, 1.0f, 1.0f);
پس مکعب مدام در حال چرخش خواهد بود.
آخرین ویرایش: